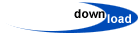 ESP32 Libraries
ESP32_DataLogger_DHT11
/* Programname: ESP32_DataLogger_DHT11/Thingspeak * Author: Lena
Jablonsky, Tobias Bruder, Jonas Messerschmid, Florian Proß *
Date: 19.11.2017 * * geändert durch : Lars Birn, Stephan Lang
* Datum : 18.04.2018 * * The circuit: * * SD card
attached to SPI bus as follows: ** GPIO 23 - MOSI ** GPIO 19 -
MISO ** GPIO 18 - CLK ** GPIO 05 - CS ** GPIO 5V - VCC //
NOT 3V3 ** GPIO GND- Ground * * I/O attached to ESP32
** GPIO 04 - Cerabar PMC21/SKU237545 analog input //????? * *
OLED display attached to I2C bus as follow ** GPIO 22 - SCL
** GPIO 21 - SDA * * Temperature Sensors ** GPIO 16 -
Data DHT1 //Auf dem Drucker ** GPIO 17 - Data DHT2 //Im Drucker
* */
//
****************************************************** DECLARATIONS
*******************************************************************
#include <SPI.h>
/////////////////////////////////////////////////////////////////
// DECLARATION WIFI/DHT
/////////////////////////////////////////////////////////////////
#include <WiFi.h> #include "DHT.h" #include <WiFiMulti.h>
// Uncomment one of the lines below for whatever DHT sensor type
you're using! #define DHTTYPE DHT11 // DHT 11 #define DHTTYPE2
DHT11
// Replace with your network credentials const
char* ssid = "OnePlus 5"; // Your SSID (Name of your WiFi) const
char* password = "5650e774bbb3"; //Your Wifi password
const
char* host = "api.thingspeak.com"; String api_key =
"RAAR3P14LNCLJBZ8"; // Your API Key provied by thingspeak
//
DHT SensorPin Declaration #define DHTPin 16 //auf dem Drucker
#define DHTPin2 17 //in dem Drucker // Initialize DHT sensors.
DHT dht(DHTPin, DHTTYPE); DHT dht2(DHTPin2, DHTTYPE2);
WiFiMulti WiFiMulti;
// Client variables char
linebuf[80]; int charcount=0;
/////////////////////////////////////////////////////////////////
// DECLARATION OLED display
/////////////////////////////////////////////////////////////////
#include <SPI.h> #include <Wire.h> #include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define OLED_RESET 4 // not
used Adafruit_SSD1306 display(OLED_RESET); //Adafruit_SSD1306
display(0x3c, 22, 21);
#define NUMFLAKES 10 #define
XPOS 0 #define YPOS 1 #define DELTAY 2
#define
LOGO16_GLCD_HEIGHT 16 #define LOGO16_GLCD_WIDTH 16 static
const unsigned char PROGMEM logo16_glcd_bmp[] = { B00000000,
B11000000, B00000001, B11000000, B00000001, B11000000,
B00000011, B11100000, B11110011, B11100000, B11111110,
B11111000, B01111110, B11111111, B00110011, B10011111,
B00011111, B11111100, B00001101, B01110000, B00011011,
B10100000, B00111111, B11100000, B00111111, B11110000,
B01111100, B11110000, B01110000, B01110000, B00000000,
B00110000 };
#if (SSD1306_LCDHEIGHT != 64)
//#error("Height incorrect, please fix Adafruit_SSD1306.h!");
#endif
/////////////////////////////////////////////////////////////////
// DECLARATIONEN Cerabar PMC21/SKU237545
/////////////////////////////////////////////////////////////////
//here we use analog pin GIO04 of ESP32 to read data #define
analogPin 4 //???????
// *** Cerabar PMC21 *** ///???????
//here we need variables to put in and calculate the value int
inputVariable0 = 0; int inputVariable1 = 0;
// ***
SKU237545 *** int SensorVal; // AD converter value float
Voltage; // Current voltage float Pressure; // Current pressure
/////////////////////////////////////////////////////////////////
// DECLARATION SD card
/////////////////////////////////////////////////////////////////
#include "FS.h" #include "SD.h" #include "SPI.h" // SPI bus
File dataFile; #define LOGDATEI "/Log" // SLASH WICHTIG für
Verzeichnis der SD Karte
/////////////////////////////////////////////////////////////////
// DECLARATION Stopwatch
/////////////////////////////////////////////////////////////////
unsigned long startzeit; unsigned long vergangeneZeit;
unsigned long zeit; int zaehlvariable = 0;
/////////////////////////////////////////////////////////////////
// DECLARATION Interrupt pin
/////////////////////////////////////////////////////////////////
// pin that is attached to interrupt // byte interruptPin = 12;
int intOccurred = -1; long debouncing_time = 1000; // Debouncing
time in milliseconds volatile unsigned long last_micros;
//// *************************************************** METHODS
***************************************************************************************
///////////////////////////////////////////////////////////////////
// // impSD() // implement SD card //
///////////////////////////////////////////////////////////////////
void impSD() { if(!SD.begin()){ Serial.println("Card Mount
Failed"); return; } uint8_t cardType = SD.cardType();
if(cardType == CARD_NONE){ Serial.println("No SD card
attached"); return; }
Serial.println("SD card
detected"); Serial.print("SD Card Type: "); if(cardType ==
CARD_MMC){ Serial.println("MMC"); } else if(cardType ==
CARD_SD){ Serial.println("SDSC"); } else if(cardType ==
CARD_SDHC){ Serial.println("SDHC"); } else {
Serial.println("UNKNOWN"); }
uint64_t cardSize =
SD.cardSize() / (1024 * 1024); Serial.printf("SD Card Size:
%lluMB\n", cardSize);
const uint8_t NAMENSLAENGE=
sizeof(LOGDATEI) - 1; String dateiName = LOGDATEI "00.csv"; //
Z.B. DHT11.csv
while (SD.exists(dateiName)) { if
(dateiName[NAMENSLAENGE + 1] != '9') { dateiName[NAMENSLAENGE
+ 1]++; } else if (dateiName[NAMENSLAENGE] != '9') {
dateiName[NAMENSLAENGE + 1] = '0'; dateiName[NAMENSLAENGE]++;
} else { Serial.println("Not able to create file -->
Log99 reached."); delay(1000); break; } } // Open
file now dataFile = SD.open(dateiName, FILE_WRITE);
Serial.println("Set-up SD card"); }
/////////////////////////////////////////////////////////////////
// // impHeader() // implement header //
/////////////////////////////////////////////////////////////////
void impHeader() { dataFile.println(F("Temp/Luftfeuchte mit
DHT11 und Druckmessung mit Cerabar PMC21/SKU237545 mit Nutzung eines
Dataloggers")); dataFile.print(F("Zeit [m]"));
dataFile.print(';'); dataFile.print(F("Druck [bar]"));
dataFile.print(';'); dataFile.print(F("Temperatur auf dem Drucker
[\tC]")); dataFile.print(';'); dataFile.print(F("Luftfeuchte
auf dem Drucker [%]")); dataFile.print(';');
dataFile.print(F("Temperatur in dem Drucker [\tC]"));
dataFile.print(';'); dataFile.print(F("Luftfeuchte in dem Drucker
[%]")); dataFile.print(';'); dataFile.println();
Serial.println("Set-up header"); }
/////////////////////////////////////////////////////////////////
// // impStoppuhr() // implement Stopwatch //
/////////////////////////////////////////////////////////////////
void impStoppuhr() { startzeit = millis(); startzeit =
startzeit/60000; Serial.print("Set-up stopwatch");
Serial.print(" Starttime of meassurement: ");
Serial.print(startzeit); Serial.print(" min");
Serial.println(); }
/////////////////////////////////////////////////////////////////
// // impOLED() // implement OLED display //
/////////////////////////////////////////////////////////////////
void impOLED() { // by default, we'll generate the high
voltage from the 3.3v line internally! (neat!)
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // initialize with the
I2C addr 0x3D (for the 128x64) // init done
// Show image
buffer on the display hardware. // Since the buffer is intialized
with an Adafruit splashscreen // internally, this will display
the splashscreen. display.display(); delay(2000);
//
Clear the buffer. display.clearDisplay();
// text display
tests display.setTextSize(1); display.setTextColor(WHITE);
display.setCursor(0,0); display.println("Informationstechnik-");
display.println("Labor:"); display.println();
display.println("3D Keramik Druck"); display.display();
delay(3000); display.clearDisplay();
Serial.println("Set-up OLED display"); }
/////////////////////////////////////////////////////////////////
// // ISR() // Interrupt service routine for reed contact
//
/////////////////////////////////////////////////////////////////
void ISR() { if((long)(micros() - last_micros) >=
debouncing_time * 1000) { Interrupt(); last_micros =
micros(); } }
void Interrupt() { intOccurred++;
Serial.println(); Serial.println("Interrupt done.");
Serial.println(); }
/////////////////////////////////////////////////////////////////
// // impInterrupt() // implement interrupt pin //
/////////////////////////////////////////////////////////////////
void impInterrupt() { pinMode(interruptPin, INPUT_PULLUP);
attachInterrupt(digitalPinToInterrupt(interruptPin), ISR, CHANGE);
Serial.println("Set-up interrupt"); }
//------------------------------------------------------------------IMPLEMENTIERUNGEN---------------------------------------------------------------------------------
///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// // setup() // initialize SD card reader, header, stopwatch,
display, // relay, Drehschalter/Poti and sensors //
///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
void setup() { Serial.begin(115200); // serial communication
on impSD(); // initialize sd card impHeader(); // initialize
header for .csv file // impStoppuhr(); // initialize stopwatch
impOLED(); // initialize OLED display impInterrupt(); //
initialize interrupt pin Connect_to_Wifi(); dht.begin();
dht2.begin();
} // Serial.println(); //
Serial.println("SET-UP DONE."); // Serial.println("");
//-------------------------------------------------------------------------MAIN--------------------------------------------------------------------------------------
///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// // Loop: // Reading sensors, print to sd, control relay
//
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
void loop() { if(intOccurred%2 == 0 && intOccurred >= 0)
{ // Includes and defines needed for putting in the SD card
while program is running #include "FS.h" #include "SD.h"
#include "SPI.h" // SPI bus
File dataFile; #define
LOGDATEI "/Log" // SLASH IMPORTANT for directory/path of SD card
dataFile.close(); Serial.println(); Serial.println("File
closed");
impSD(); // initialize sd card impHeader(); //
initialize header for .csv file impStoppuhr(); // initialize
stopwatch
intOccurred = -2; }
if (WiFi.status() !=
WL_CONNECTED){ Connect_to_Wifi(); } Serial.print("Loop for
new meassurement");
// Get time zeit = getTime();
float h = dht.readHumidity(); //auf dem Drucker pin 16 h = h + 9;
//Offset float t = dht.readTemperature(); //auf dem Drucker t
= t - 2;
//Offset if (h>=99){ h = 99; } delay(2000); float h2
= dht2.readHumidity(); //in dem Drucker pin 17 h2 = h2 + 6;
//Offset float t2 = dht2.readTemperature(); //in dem Drucker
t2 = t2 - 1;
//Offset if (h2>=99){ h2 = 99; } delay (3000); //Get
pressure value from Cerabar PMC21/SKU237545 Pressure =
getPressure();
// Print to seriell monitor
//seriellAusgabe();
// Print to OLED display
OLED(t,h,t2,h2);
if(zaehlvariable%7 == 0){ //send to
Thingspeak Send_Data(t, t2, h, h2, Pressure);
Serial.println("gesendet an Homepage"); } /* Write data to sd
card depends how modulo is set: %40 --> 1 min %200 --> 5 min
*/ if(zaehlvariable%7 == 0) { schreibeMessung(zeit,
Pressure, t, h, t2, h2); Serial.println("Print SD card done");
} zaehlvariable++; }
/////////////////////////////////////////////////////////////////
// // Send_Data // Sendet Daten zu Thingspeak //
/////////////////////////////////////////////////////////////////
void Send_Data(float t, float t2, float h, float h2, float pressure)
{
Serial.println("Prepare to send data");
// Use
WiFiClient class to create TCP connections WiFiClient client;
const int httpPort = 80;
if (!client.connect(host,
httpPort)) { Serial.println("connection failed"); return; }
else { String data_to_send = api_key; data_to_send +=
"&field1="; data_to_send += String(t); data_to_send +=
"&field2="; data_to_send += String(h); data_to_send +=
"&field3="; data_to_send += String(t2); data_to_send +=
"&field4="; data_to_send += String(h2); data_to_send +=
"&field5="; data_to_send += String(pressure); data_to_send +=
"\r\n\r\n";
client.print("POST /update HTTP/1.1\n");
client.print("Host: api.thingspeak.com\n");
client.print("Connection: close\n");
client.print("X-THINGSPEAKAPIKEY: " + api_key + "\n");
client.print("Content-Type: application/x-www-form-urlencoded\n");
client.print("Content-Length: ");
client.print(data_to_send.length()); client.print("\n\n");
client.print(data_to_send); }
client.stop();
}
/////////////////////////////////////////////////////////////////
// // Connect_to_Wifi // Verbindung mit WLAN herstellen //
/////////////////////////////////////////////////////////////////
void Connect_to_Wifi(){ Serial.println();
Serial.println(); Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, password);
//
attempt to connect to Wifi network: while(WiFi.status() !=
WL_CONNECTED) { // Connect to WPA/WPA2 network. Change this line
if using open or WEP network: delay(500); Serial.print(".");
} Serial.println(""); Serial.println("WiFi connected");
Serial.println("IP address: "); Serial.println(WiFi.localIP());
}
/////////////////////////////////////////////////////////////////
// // schreibeMessung() // Write messurement values to SD card
//
/////////////////////////////////////////////////////////////////
void schreibeMessung(unsigned long vergZeit, float pressure, float
temperature, float humidity, float t2, float h2) {
dataFile.print(vergZeit); dataFile.print(';');
dataFile.print(pressure); dataFile.print(';');
dataFile.print(temperature); dataFile.print(';');
dataFile.print(humidity); dataFile.print(';');
dataFile.print(t2); dataFile.print(';'); dataFile.print(h2);
dataFile.println(); Serial.println("auf SD-Karte"); }
/////////////////////////////////////////////////////////////////
// // seriellAusgabe() // Print to serial monitor //
/////////////////////////////////////////////////////////////////
//int seriellAusgabe(float t,float t2,float h ,float h2) {
Serial.print("Humidity: "); Serial.print(h); Serial.print("
%\t Temperature: "); Serial.print(t); Serial.print(" *C ");
Serial.print("Humidity: "); Serial.print(h2); Serial.print("
%\t Temperature: "); Serial.print(t2); }
/////////////////////////////////////////////////////////////////
// // OLED() // Print to OLED display //
/////////////////////////////////////////////////////////////////
int OLED(float t, float h, float t2, float h2) {
display.setTextSize(1); display.setTextColor(WHITE);
display.setCursor(0,0); display.print("Temperatur: (auf/in)");
display.println(); display.print(t); display.print(" / ");
display.print(t2); display.println(" \tC");
display.print("Luftfeuchtigkeit: "); display.println();
display.print(h); display.print(" / "); display.print(h2);
display.println(" %"); display.display();
display.clearDisplay(); Serial.println("OLED"); }
/////////////////////////////////////////////////////////////////
// // Zeit() // get the time //
/////////////////////////////////////////////////////////////////
unsigned long getTime() { vergangeneZeit = millis() -
startzeit; vergangeneZeit = vergangeneZeit/60000; //
Serial.println(); // Serial.print("Messung nach: "); //
Serial.print(vergangeneZeit); // Serial.print(" min"); //
Serial.println(); return vergangeneZeit; }
/////////////////////////////////////////////////////////////////
// // pressure() // Read pressure from Cerabar PMC21/SKU237545
via analog in //
/////////////////////////////////////////////////////////////////
int getPressure() { // *** Cerabar PMC21 *** //
inputVariable0 = 0; // This value must be cleared before next
reading // // // Lesen // inputVariable1 =
analogRead(analogPin); // Reading pressure sensor via analog input
-> 12 bit AD converter (4096) // inputVariable0 = inputVariable0
+ inputVariable1; // inputVariable0 = (inputVariable0 - 2048) /
818 ; // Calcaulate the value of AD converter to voltage // //
// Ausgabe auf Konsole // Serial.print("Pressure: "); //
Serial.print(inputVariable0); // Serial.println(" bar"); //
// return inputVariable0;
// Reading pressure sensor
via analog input -> 12 bit AD converter (4096) int SensorVal =
analogRead(analogPin); //Serial.print(SensorVal);
//
Calcaulate the value of AD converter to voltage float Voltage =
(SensorVal/4096.0)*3.3; // 0 bar = 215 (sensorVal) // 6 bar =
2048 (sensorVal) // 12 bar = 4096 (sensorVal)
// Calculate
the value of the voltage to bar float Pressure =
(Voltage-0.18)*4.65;
return Pressure; }
// ***
SKU237545 *** // // // Reading pressure sensor via analog
input -> 12 bit AD converter (4096) // int SensorVal =
analogRead(analogPin); // Serial.print(SensorVal); // // //
Calcaulate the value of AD converter to voltage // float Voltage
= (SensorVal/4096.0)*3.3; // 0 bar = 215 (sensorVal) // // 6 bar
= 2048 (sensorVal) // // 12 bar = 4096 (sensorVal) // // //
Calculate the value of the voltage to bar // float Pressure =
(Voltage-0.18)*4.65; // // return Pressure; //} |